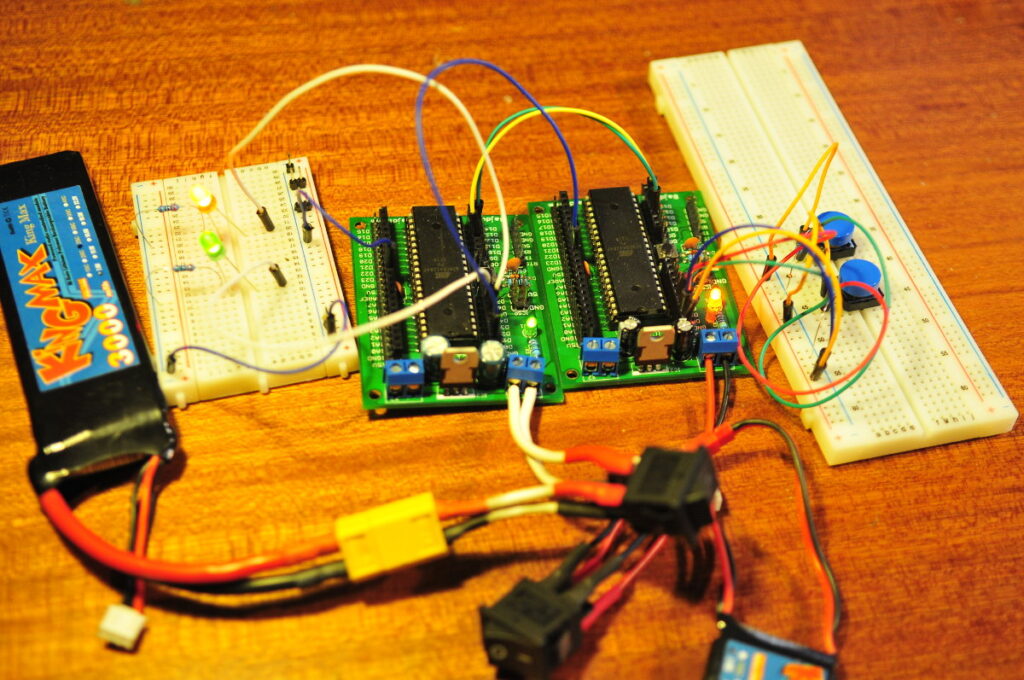
Arduino serial communication
While I was searching for information on how to establish communication between 2 Arduino’s over the I2C bus I stumbled on Bill Porters website. He has written an Arduino library for easy serial communication between 2 Arduino’s. And someone else has made a version of the same library for easy I2C communication.
The EasyTransfer serial library has 2 versions, one for hardware serial and one for software serial. The libraries can be found on github. Today I tried out the EasyTranfer library and used it with the hardware serial ports of 2 ATmega1284’s. The ATmega1284 has 2 serial ports so while you can use one serial port for the serial monitor you can use the other serial port for communication with another micro controller.
I connected 2 push buttons to one ATmega1284 and 2 leds to the other ATmega. For serial communication you must connect RX to TX and TX to RX. Both micro controllers must also share the same ground. In the following sketches the state of the push button is sent to the other micro controller over the serial connection. I used the bounce library to debounce the push buttons. The EasyTransfer library is indeed easy to use for simple serial communication 🙂 I only tried one way communication but the library allows communication in both directions.
The transmitter (TX) sketch:
// http://www.bajdi.com // TX serial communication with the EasyTransfer library // This sketch sends the state of the 2 push buttons using serial 2 // The sketch will only work with an Arduino Mega unless you change the serial2 to serial #include <EasyTransfer.h> #include <Bounce.h> //create object EasyTransfer ET; struct SEND_DATA_STRUCTURE{ //put your variable definitions here for the data you want to send //THIS MUST BE EXACTLY THE SAME ON THE OTHER ARDUINO int led1State; int led2State; }; const int button1 = 0; // the number of the first pushbutton pin const int button2 = 1; // the number of the first pushbutton pin int lastButton1State = LOW; // the previous reading from the first push button pin int lastButton2State = LOW; // the previous reading from the second push button pin Bounce bouncer1 = Bounce(button1,50); //setting up the debouncing for the first push button Bounce bouncer2 = Bounce(button2,50); //setting up the debouncing for the second push button //give a name to the group of data SEND_DATA_STRUCTURE mydata; void setup(){ Serial1.begin(115200); pinMode(button1, INPUT); pinMode(button2, INPUT); //start the library, pass in the data details and the name of the serial port. Can be Serial, Serial1, Serial2, etc. ET.begin(details(mydata), &Serial1); } void loop(){ bouncer1.update ( ); // Update the debouncer for the first push button bouncer2.update ( ); // Update the debouncer for the second push button int reading1 = bouncer1.read(); // Get the update value int reading2 = bouncer2.read(); // Get the update value reading1 == HIGH; reading2 == HIGH; if (lastButton1State == LOW && reading1 == HIGH) { if (mydata.led1State == HIGH) {mydata.led1State = LOW;} else {mydata.led1State = HIGH;} } lastButton1State = reading1; if (lastButton2State == LOW && reading2 == HIGH) { if (mydata.led2State == HIGH) {mydata.led2State = LOW;} else {mydata.led2State = HIGH;} } lastButton2State = reading2; ET.sendData(); delay(150); }
The receiver (RX) sketch:
// http://www.bajdi.com // RX serial communication with the EasyTransfer library // This sketch receives the state of 2 push buttons using serial 2 and lights up 2 leds // The sketch will only work with an Arduino Mega unless you change the serial2 to serial #include <EasyTransfer.h> //create object EasyTransfer ET; const int led1 = 3; // the number of the LED1 pin const int led2 = 4; // the number of the LED2 pin struct RECEIVE_DATA_STRUCTURE{ //put your variable definitions here for the data you want to receive //THIS MUST BE EXACTLY THE SAME ON THE OTHER ARDUINO int led1State; int led2State; }; //give a name to the group of data RECEIVE_DATA_STRUCTURE mydata; void setup(){ Serial1.begin(115200); //start the library, pass in the data details and the name of the serial port. Can be Serial, Serial1, Serial2, etc. ET.begin(details(mydata), &Serial1); pinMode(led1, OUTPUT); pinMode(led2, OUTPUT); } void loop(){ //check and see if a data packet has come in. if(ET.receiveData()){ //this is how you access the variables. [name of the group].[variable name] //since we have data, we will blink it out. if (mydata.led1State == HIGH) { digitalWrite(led1, HIGH); } else { digitalWrite(led1, LOW); } if (mydata.led2State == HIGH) { digitalWrite(led2, HIGH); } else { digitalWrite(led2, LOW); } } //you should make this delay shorter then your transmit delay or else messages could be lost delay(50); }