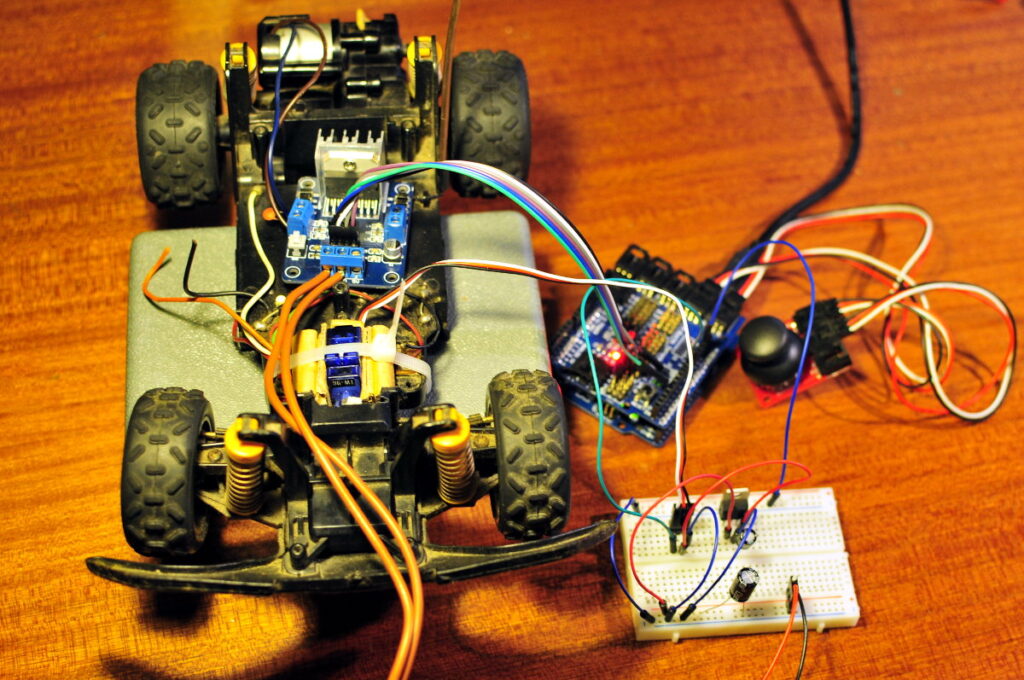
Arduino RC car
Fiddled some more with my old Nikko rc car, last time I managed to control the main motor with a L298n motor controller board. Since my last post about it I found out that the servo that originally controlled the front wheels is broken. So I needed to find a solution for that. I had a small Tower Pro SG90 servo and decided to fit that. With the use of my Dremel, tie wrap, a small bolt and pieces of carton I succeeded in mounting the servo so it can turn the front wheels 🙂 To be honest it took me a couple of hours and a lot of swearing to get it right.
So after mounting the servo it was time to connect it all to an Arduino. I connected the L298n board, SG90 servo and joystick to an Arduino Duemilanove to test if everything worked like it should. I used my lab power supply to power everything, I gave 7-8V to the L298n and used a L7805CV to give the servo 5V, I think 7-8V is a bit to much for these little servos. I actually first tried to power the servo from the Arduino, but it would just reset every time I tried to move the servo.
I made the following sketch that lets me control the main motor and front wheels with an analog joystick. Next step is to control it wireless with my self made remote control using a pair of nRF24L01 modules.
/* http://www.bajdi.com RC car controlled by analog joystick Micro SG90 servo to turn front wheels DC motor controlled by L298n */ #include <Servo.h> Servo myservo; // create servo object to control a servo int Y; // Y axis = forward/backward int X; // X axis = left/right int fspeed; // forward speed int bspeed; // backward speed int iOldPos, iNewPos = 0; // servo position const int in1 = 2; // direction pin 1 const int in2 = 4; // direction pin 2 const int ena = 3; // PWM pin to change speed long previousMillis = 0; // timer for servo const int interval = 30; // interval to update servo position void setup() { pinMode(in1, OUTPUT); // connection to L298n pinMode(in2, OUTPUT); // connection to L298n pinMode(ena, OUTPUT); // connection to L298n myservo.attach(9); // attaches the servo on pin 9 to the servo object myservo.write(87); // center servo delay(50); // give servo time to change position } void loop() { int X = analogRead(A1); // joystick X axis int Y = analogRead(A0); // joystick Y axis if (Y < 500) // joystick forward { fspeed = (map(Y, 501, 0, 70, 250)); forward(fspeed); } if (Y > 540) // joystick backward { bspeed = (map(Y, 541, 1023, 70, 250)); backward(bspeed); } if (Y >= 500 && Y <= 540) // joystick is centered { stop(); } if (X > 490 && X < 530 ) // joystick is centered { iNewPos = 87; // 87 = servo in center position } if (X < 490) { iNewPos = (map(X, 491, 0, 88, 130)); } if (X > 530) { iNewPos = (map(X, 531, 1023, 86, 35)); } unsigned long currentMillis = millis(); if(iOldPos != iNewPos && currentMillis - previousMillis > interval) { // Issue command only if desired position changes and interval is over previousMillis = currentMillis; iOldPos = iNewPos; myservo.write(iNewPos); // tell servo to go to position in variable 'pos' } } void stop() { analogWrite(ena, 0); digitalWrite(in1, LOW); digitalWrite(in2, LOW); } void forward(int fspeed) { digitalWrite(in1, HIGH); digitalWrite(in2, LOW); analogWrite(ena, fspeed); } void backward(int bspeed) { digitalWrite(in1, LOW); digitalWrite(in2, HIGH); analogWrite(ena, bspeed); }
- Arduino RC car
- Arduino RC car
- Arduino RC car
- Arduino RC car
[…] again It wasn’t easy to be honest, there were several obstacles along the way. Since my last post I had found out that the L298 H-bridge was not powerful enough to drive the motor. It would quickly […]
Hello Badji,
Your code is runnig very good. Thanks. So i have question. How can up motor speed ? Because my motor is strong but these settings remains slow.
Use a battery with a higher voltage and that can supply more current.
i have a external power supply and i use it. Motor is slowly.